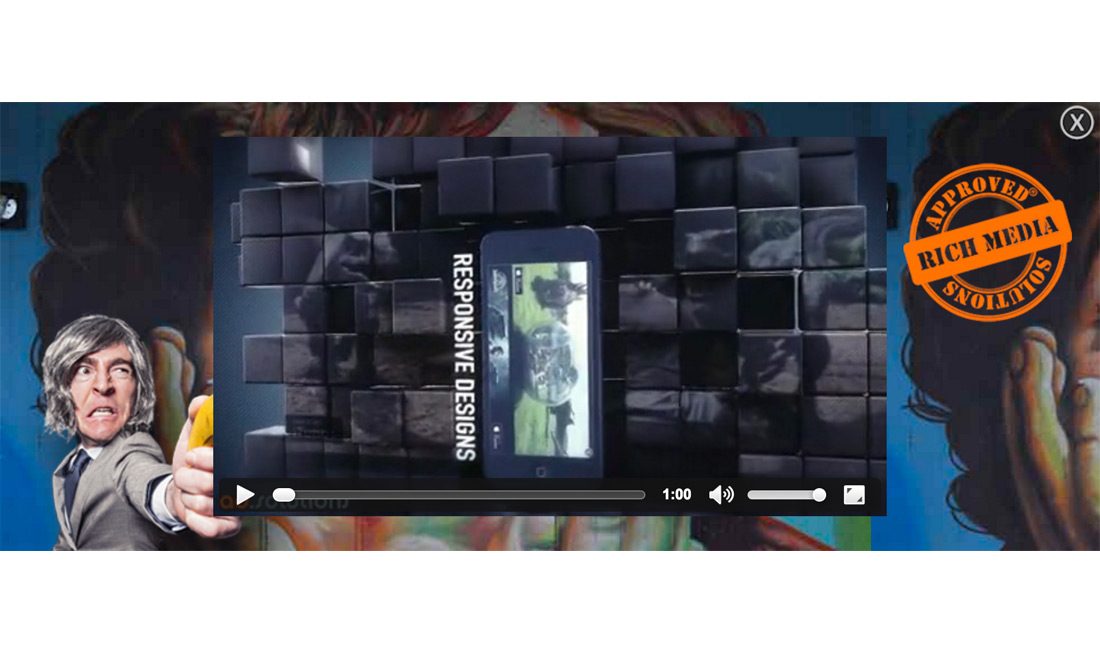
PUSHDOWN DOCUMENTATION
The templates are made as basic as possible so you have a simple starting point to begin building your creative.
The template consists of the following assets:
- index.html
- code.js
- style.css
index.html
The head of the html contains the ad size and links to the core library and other two template assets.
<!doctype html> <html> <head> <meta name="ad.size" content="width=980,height=400"> <meta name="viewport" content="user-scalable=no, width=device-width, initial-scale=1.0, maximum-scale=1.0"> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <link rel="stylesheet" type="text/css" href="main.css"> <script type="text/javascript" src="http://onecreative.aol.com/ads/jsapi/ADTECH.js"></script> <script type="text/javascript" src="code.js" async></script> <title></title> </head>
The body contains a background div and a standard html video player with an ID. You can add your own sources and change the controls etc.
<body> <div id="background" class=""> <div id="videoContainer" class="hide"> <video id="video" poster="images/video.jpg" preload="auto" controls> <source src="video.mp4" type="video/mp4"/> <source src="video.webmsd.webm" type="video/webm"/> </video> </div> <div id="closeButton" class="button hide"></div> <div id="expandButton" class="button"></div> </div> </body>
code.js
The code is wrapped within the init() function. This function is called when our library is ready.
The highlighted lines show we look for an element with ID videoPlayer (the ID of the video element) and the next highlighted line we tell the library to register this element as the video player. This way we can measure the VAST video events and report on them.
// JavaScript Document function init() { var background = document.getElementById("background"); var expandButton = document.getElementById('expandButton'); var closeButton = document.getElementById('closeButton'); var videoContainer = document.getElementById('videoContainer'); var vid = document.getElementById('video'); var contracted = true; var firstPlay = false; var isPlaying = false; //// Register the video player element to enable video event measurement //// ADTECH.registerVideoPlayer(vid);
Next a bunch of general functions. Their purpose is explained in the comments of the file but we’ll go by them one by one.
The prefixedEvent function adds browser prefixes. (element, type, callback). Further down the code you can find a sample prefixedEvent(background, “AnimationEnd”, contractHandler);
//// General function to add browser prefix //// } var pfx = ["webkit", "moz", "ms", "o", "khtml", ""]; function prefixedEvent(element, type, callback) { for (var p = 0; p < pfx.length; p++) { if (!pfx[p]) type = type.toLowerCase(); element.addEventListener(pfx[p] + type, callback, false); } }
The stopPropagation function prevents further propagation of the current event in the capturing and bubbling phases for several browsers.
//// General function to cancel bubble //// function stopPropagation(e) { if (e.stopPropagation) { e.stopPropagation(); } else { e.returnValue = false; e.cancelBubble = true; } }
The addClass allows you to add a class. addClass(elm, clsName);
//// General function that can add a class //// function addClass(elm, clsName){ if(typeof(elm) != 'array'){ elm = [elm]; } for (var i = 0; i < elm.length; i++) { if (elm[i].classList) { elm[i].classList.add(clsName); } else { if (elm[i].className.match(new RegExp('?:^|\s)' + clsName + '(?!\S)'))) { elm[i].className = elm[i].className.replace(new RegExp('?:^|\s)' + clsName + '(?!\S)'), ""); } }} }
The hideElement function can hide an element. It calls the addClass function with the ‘hide’ class Further down the code you can see some examples:
hideElement(closeButton);
hideElement(videoContainer);
Adds the class ‘hide’ to both the closeButton and the videoContainer elements.
//// General function that calls the addClass() function with a fixed class 'hide' //// function hideElement(elm){ addClass(elm,'hide'); }
The opposite of the addClass function. The removeClass function can remove a class. removeClass(elm, clsName);
//// General function that can remove a class //// function removeClass(elm, clsName) { if(typeof(elm) != 'array'){ elm = [elm]; } for(var i=0;i<elm.length;i++){ if (elm[i].classList) { elm[i].classList.remove(clsName); } else { if (elm[i].className.match(new RegExp('?:^|\s)' + clsName + '(?!\S)'))) { elm[i].className = elm[i].className.replace(new RegExp('?:^|\s)' + clsName + '(?!\S)'), ""); } }} }
The showElement function can unhide an element. It calls the removeClass function with the ‘hide’ class. Further down the code you can see some examples:
showElement(closeButton);
showElement(videoContainer);
Removes the class ‘hide’ to both the closeButton and the videoContainer elements. Making them visible again.
//// General function that calls the removeClass() function and specifically removes the 'hide' class //// function showElement(elm){ removeClass(elm,'hide'); }
Next a vid.onended function. It’s currently empty but if you want to make something happen when the video ends playback you can put it here.
Next the closeButton handler. It manages what happens when the close button is clicked. You can see that also calls the contractHandler on AnimationEnd of the background element. This is the next function.
//// Click handler for the close buutton. Tells the system the ad needs to start contracting //// closeButton.onclick = function (e) { e = getEventObject(e); prefixedEvent(background, "AnimationEnd", contractHandler); ADTECH.event('Contract Start'); contracted = true; hideElement(closeButton); hideElement(videoContainer); background.className = "contracted"; expandButton.style.display = "block"; stopPropagation(e); };
Then the contractHandler function. The highlighted line is mandatory and is there to tell our system we need to contract the iframe in which the ad is rendered.
//// Contract handler. Tells the system the ad has fully contracted //// function contractHandler(e) { if (e.animationName == "contract") { ADTECH.contract(); ADTECH.event('Contract Finished'); } }
Next the expandButton function. It handles what happens when the expandButton element is clicked. The highlighted line is mandatory and is there to tell our system we need to expand the iframe in which the ad is rendered.
//// Click handler for the expand button. Tells the system the ad needs to start expanding //// expandButton.onclick = function (e) { e = getEventObject(e); prefixedEvent(background, "AnimationEnd", expandHandler); if (contracted) { ADTECH.expand(); ADTECH.event('Expand Start'); } expandButton.style.display = "none"; background.className = " expanded"; contracted = false; };
Then the expandHandler function which triggers an event and shows the close button and video container.
//// Expand handler. Tells the system the ad has fully expanded //// function expandHandler(e) { if (e.animationName == "expand") { ADTECH.event('Expand Finished'); showElement(closeButton); showElement(videoContainer); } }
The code.js file is also the spot where the click is implemented. You can change the URL in this file. Or designate it to a different element or add multiple clickable elements.
//// Click handler //// background.onclick = function (e) { e = getEventObject(e); var obj = e.target || e.srcElement; if (!contracted && obj.className.indexOf("button") == -1) { /// add your "name" and "click URL" here /// ADTECH.click("expanded", "http://www.adsolutions.com"); } };
The click is syntax is like so: ADTECH.click(‘Click Name‘, ‘your CLICK URL‘);
Click Name is used in the reports to distinguish which click through button was clicked on. You don’t have to enter the URL if you don’t know it yet. We can handle it for you if you send the URL or redirect later on.
SUPPORT
During the design phase we can provide you with a preview URL so you can see the work in action and make changes to your design accordingly. Send us the source files and fonts to [email protected] (WeTransfer preferred)
Final creative or requests for a preview can be send to [email protected]
Also read the GENERAL DOCUMENTATION fur further reference on building HTML5 ads.